旋转(Rotation)
在本章中,我们向您解释了如何使用JOGL旋转对象。 使用GLMatrixFunc接口的glRotatef(float angle, float x, float y, float z)方法,可以沿三个轴中的任意一个旋转对象。 您需要将旋转角度和x,y,z轴作为参数传递给此方法。
以下步骤指导您成功旋转对象 -
最初使用gl.glClear(GL2.GL_COLOR_BUFFER_BIT | GL2.GL_DEPTH_BUFFER_BIT)方法清除颜色缓冲区和深度缓冲区。 此方法擦除对象的先前状态并使视图清晰。
使用glLoadIdentity()方法重置投影矩阵。
实例化animator类并使用start()方法启动动画师。
FPSAnimator类
下面给出了FPSAnimator类的各种构造函数。
Sr.No. | 方法和描述 |
---|---|
1 | FPSAnimator(GLAutoDrawable drawable, int fps) 它创建了一个FPSAnimator,它具有给定的每秒帧数值和一个初始可绘制的动画。 |
2 | FPSAnimator(GLAutoDrawable drawable, int fps, boolean cheduleAtFixedRate) 它创建一个FPSAnimator,它具有给定的目标帧每秒值,一个初始可绘制的动画,以及一个指示是否使用固定速率调度的标志。 |
3 | FPSAnimator(int fps) 它创建一个具有给定目标帧每秒值的FPSAnimator。 |
4 | 它创建一个具有给定目标帧每秒值的FPSAnimator和一个指示是否使用固定速率调度的标志。 |
它创建一个具有给定目标帧每秒值的FPSAnimator和一个指示是否使用固定速率调度的标志。
start()和stop()是这个类中的两个重要方法。 以下程序显示如何使用FPSAnimator类旋转三角形 -
import javax.media.opengl.GL2;
import javax.media.opengl.GLAutoDrawable;
import javax.media.opengl.GLCapabilities;
import javax.media.opengl.GLEventListener;
import javax.media.opengl.GLProfile;
import javax.media.opengl.awt.GLCanvas;
import javax.swing.JFrame;
import com.jogamp.opengl.util.FPSAnimator;
public class TriangleRotation implements GLEventListener {
private float rtri; //for angle of rotation
@Override
public void display( GLAutoDrawable drawable ) {
final GL2 gl = drawable.getGL().getGL2();
gl.glClear (GL2.GL_COLOR_BUFFER_BIT | GL2.GL_DEPTH_BUFFER_BIT );
// Clear The Screen And The Depth Buffer
gl.glLoadIdentity(); // Reset The View
//triangle rotation
gl.glRotatef( rtri, 0.0f, 1.0f, 0.0f );
// Drawing Using Triangles
gl.glBegin( GL2.GL_TRIANGLES );
gl.glColor3f( 1.0f, 0.0f, 0.0f ); // Red
gl.glVertex3f( 0.5f,0.7f,0.0f ); // Top
gl.glColor3f( 0.0f,1.0f,0.0f ); // blue
gl.glVertex3f( -0.2f,-0.50f,0.0f ); // Bottom Left
gl.glColor3f( 0.0f,0.0f,1.0f ); // green
gl.glVertex3f( 0.5f,-0.5f,0.0f ); // Bottom Right
gl.glEnd();
gl.glFlush();
rtri += 0.2f; //assigning the angle
}
@Override
public void dispose( GLAutoDrawable arg0 ) {
//method body
}
@Override
public void init( GLAutoDrawable arg0 ) {
// method body
}
@Override
public void reshape( GLAutoDrawable drawable, int x, int y, int width, int height ) {
public static void main( String[] args ) {
//getting the capabilities object of GL2 profile
final GLProfile profile = GLProfile.get(GLProfile.GL2 );
GLCapabilities capabilities = new GLCapabilities( profile );
// The canvas
final GLCanvas glcanvas = new GLCanvas( capabilities);
TriangleRotation triangle = new TriangleRotation();
glcanvas.addGLEventListener( triangle );
glcanvas.setSize( 400, 400 );
// creating frame
final JFrame frame = new JFrame ("Rotating Triangle");
// adding canvas to it
frame.getContentPane().add( glcanvas );
frame.setSize(frame.getContentPane() .getPreferredSize());
frame.setVisible( true );
//Instantiating and Initiating Animator
final FPSAnimator animator = new FPSAnimator(glcanvas, 300,true);
animator.start();
}
} //end of main
} //end of class
如果编译并执行上述程序,则会生成以下输出。 在这里,您可以观察围绕x轴旋转彩色三角形的各种快照。
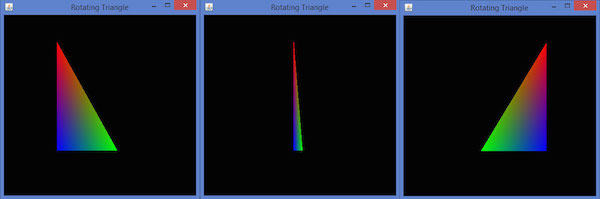