服务定位器模式(Service Locator Pattern)
当我们想要使用JNDI查找定位各种服务时,使用服务定位器设计模式。 考虑到为服务查找JNDI的高成本,Service Locator模式使用缓存技术。 第一次需要服务时,Service Locator在JNDI中查找并缓存服务对象。 通过Service Locator进行进一步查找或相同的服务在其缓存中完成,这在很大程度上提高了应用程序的性能。 以下是此类设计模式的实体。
Service - 将处理请求的实际服务。 在JNDI服务器中查看此类服务的引用。
Context/Initial Context - JNDI上下文携带用于查找目的的服务的引用。
Service Locator - 服务定位器是通过JNDI查找缓存服务来获取服务的单一联系点。
Cache - 用于存储服务引用的缓存以重用它们
Client - 客户端是通过ServiceLocator调用服务的对象。
实现 (Implementation)
我们将创建一个ServiceLocator , InitialContext , Cache , Service作为代表我们实体的各种对象。 Service1和Service2代表具体服务。
ServiceLocatorPatternDemo ,我们的演示类,在这里充当客户端,并将使用ServiceLocator来演示服务定位器设计模式。
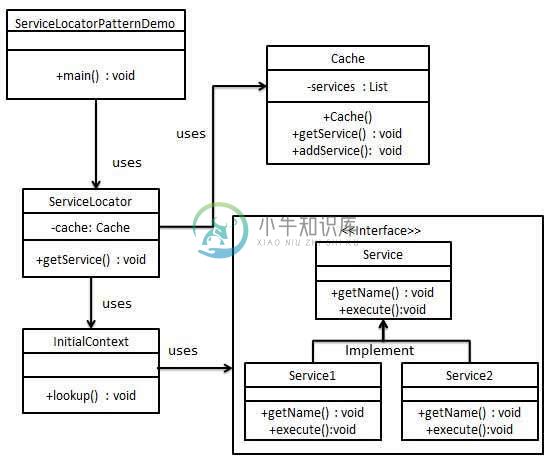
Step 1
创建服务界面。
Service.java
public interface Service {
public String getName();
public void execute();
}
Step 2
创建具体服务。
Service1.java
public class Service1 implements Service {
public void execute(){
System.out.println("Executing Service1");
}
@Override
public String getName() {
return "Service1";
}
}
Service2.java
public class Service2 implements Service {
public void execute(){
System.out.println("Executing Service2");
}
@Override
public String getName() {
return "Service2";
}
}
Step 3
为JNDI查找创建InitialContext
InitialContext.java
public class InitialContext {
public Object lookup(String jndiName){
if(jndiName.equalsIgnoreCase("SERVICE1")){
System.out.println("Looking up and creating a new Service1 object");
return new Service1();
}
else if (jndiName.equalsIgnoreCase("SERVICE2")){
System.out.println("Looking up and creating a new Service2 object");
return new Service2();
}
return null;
}
}
Step 4
创建缓存
Cache.java
import java.util.ArrayList;
import java.util.List;
public class Cache {
private List<Service> services;
public Cache(){
services = new ArrayList<Service>();
}
public Service getService(String serviceName){
for (Service service : services) {
if(service.getName().equalsIgnoreCase(serviceName)){
System.out.println("Returning cached " + serviceName + " object");
return service;
}
}
return null;
}
public void addService(Service newService){
boolean exists = false;
for (Service service : services) {
if(service.getName().equalsIgnoreCase(newService.getName())){
exists = true;
}
}
if(!exists){
services.add(newService);
}
}
}
Step 5
创建服务定位器
ServiceLocator.java
public class ServiceLocator {
private static Cache cache;
static {
cache = new Cache();
}
public static Service getService(String jndiName){
Service service = cache.getService(jndiName);
if(service != null){
return service;
}
InitialContext context = new InitialContext();
Service service1 = (Service)context.lookup(jndiName);
cache.addService(service1);
return service1;
}
}
Step 6
使用ServiceLocator演示服务定位器设计模式。
ServiceLocatorPatternDemo.java
public class ServiceLocatorPatternDemo {
public static void main(String[] args) {
Service service = ServiceLocator.getService("Service1");
service.execute();
service = ServiceLocator.getService("Service2");
service.execute();
service = ServiceLocator.getService("Service1");
service.execute();
service = ServiceLocator.getService("Service2");
service.execute();
}
}
Step 7
验证输出。
Looking up and creating a new Service1 object
Executing Service1
Looking up and creating a new Service2 object
Executing Service2
Returning cached Service1 object
Executing Service1
Returning cached Service2 object
Executing Service2