Building RESTful Web Services
本章将详细讨论如何使用jQuery AJAX来使用RESTful Web服务。
创建一个简单的Spring Boot Web应用程序并编写一个控制器类文件,用于重定向到HTML文件以使用RESTful Web服务。
我们需要在构建配置文件中添加Spring Boot启动程序Thymeleaf和Web依赖项。
对于Maven用户,请在pom.xml文件中添加以下依赖项。
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
对于Gradle用户,将以下依赖项添加到build.gradle文件中 -
compile group: ‘org.springframework.boot’, name: ‘spring-boot-starter-thymeleaf’
compile(‘org.springframework.boot:spring-boot-starter-web’)
@Controller类文件的代码如下 -
@Controller
public class ViewController {
}
您可以定义请求URI方法以重定向到HTML文件,如下所示 -
@RequestMapping(“/view-products”)
public String viewProducts() {
return “view-products”;
}
@RequestMapping(“/add-products”)
public String addProducts() {
return “add-products”;
}
此API http://localhost:9090/products应返回以下JSON作为响应,如下所示 -
[
{
"id": "1",
"name": "Honey"
},
{
"id": "2",
"name": "Almond"
}
]
现在,在类路径的templates目录下创建一个view-products.html文件。
在HTML文件中,我们添加了jQuery库并编写了代码以在页面加载时使用RESTful Web服务。
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$.getJSON("http://localhost:9090/products", function(result){
$.each(result, function(key,value) {
$("#productsJson").append(value.id+" "+value.name+" ");
});
});
});
</script>
POST方法和此URL http://localhost:9090/products应包含以下Request Body和Response正文。
请求正文的代码如下 -
{
"id":"3",
"name":"Ginger"
}
Response body的代码如下 -
Product is created successfully
现在,在类路径的templates目录下创建add-products.html文件。
在HTML文件中,我们添加了jQuery库,并在单击按钮时编写了将表单提交到RESTful Web服务的代码。
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script>
$(document).ready(function() {
$("button").click(function() {
var productmodel = {
id : "3",
name : "Ginger"
};
var requestJSON = JSON.stringify(productmodel);
$.ajax({
type : "POST",
url : "http://localhost:9090/products",
headers : {
"Content-Type" : "application/json"
},
data : requestJSON,
success : function(data) {
alert(data);
},
error : function(data) {
}
});
});
});
</script>
完整的代码如下。
Maven - pom.xml文件
<?xml version = ”1.0” encoding = ”UTF-8”?>
<project xmlns = ”http://maven.apache.org/POM/4.0.0”
xmlns:xsi = ”http://www.w3.org/2001/XMLSchema-instance”
xsi:schemaLocation = ”http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd”>
<modelVersion>4.0.0</modelVersion>
<groupId>cn.xnip</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.8.RELEASE</version>
<relativePath />
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Gradle - build.gradle的代码如下 -
buildscript {
ext {
springBootVersion = ‘1.5.8.RELEASE’
}
repositories {
mavenCentral()
}
dependencies {
classpath(“org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}”)
}
}
apply plugin: ‘java’
apply plugin: ‘eclipse’
apply plugin: ‘org.springframework.boot’
group = ‘cn.xnip’
version = ‘0.0.1-SNAPSHOT’
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile(‘org.springframework.boot:spring-boot-starter-web’)
compile group: ‘org.springframework.boot’, name: ‘spring-boot-starter-thymeleaf’
testCompile(‘org.springframework.boot:spring-boot-starter-test’)
}
下面给出的控制器类文件 - ViewController.java如下 -
package cn.xnip.demo.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class ViewController {
@RequestMapping(“/view-products”)
public String viewProducts() {
return “view-products”;
}
@RequestMapping(“/add-products”)
public String addProducts() {
return “add-products”;
}
}
view-products.html文件如下 -
<!DOCTYPE html>
<html>
<head>
<meta charset = "ISO-8859-1"/>
<title>View Products</title>
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$.getJSON("http://localhost:9090/products", function(result){
$.each(result, function(key,value) {
$("#productsJson").append(value.id+" "+value.name+" ");
});
});
});
</script>
</head>
<body>
<div id = "productsJson"> </div>
</body>
</html>
add-products.html文件如下 -
<!DOCTYPE html>
<html>
<head>
<meta charset = "ISO-8859-1" />
<title>Add Products</title>
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script>
$(document).ready(function() {
$("button").click(function() {
var productmodel = {
id : "3",
name : "Ginger"
};
var requestJSON = JSON.stringify(productmodel);
$.ajax({
type : "POST",
url : "http://localhost:9090/products",
headers : {
"Content-Type" : "application/json"
},
data : requestJSON,
success : function(data) {
alert(data);
},
error : function(data) {
}
});
});
});
</script>
</head>
<body>
<button>Click here to submit the form</button>
</body>
</html>
主要的Spring Boot Application类文件如下 -
package cn.xnip.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
现在,您可以创建可执行的JAR文件,并使用以下Maven或Gradle命令运行Spring Boot应用程序。
对于Maven,请使用下面给出的命令 -
mvn clean install
在“BUILD SUCCESS”之后,您可以在目标目录下找到JAR文件。
对于Gradle,请使用下面给出的命令 -
gradle clean build
在“BUILD SUCCESSFUL”之后,您可以在build/libs目录下找到JAR文件。
使用以下命令运行JAR文件 -
java –jar <JARFILE>
现在,应用程序已在Tomcat端口8080上启动。

现在点击Web浏览器中的URL,您可以看到如下所示的输出 -
HTTP://本地主机:8080 /视图副产物
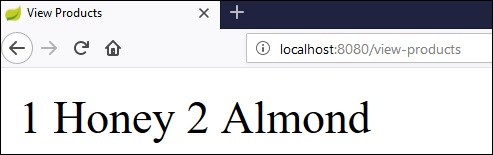
HTTP://本地主机:8080 /附加产品
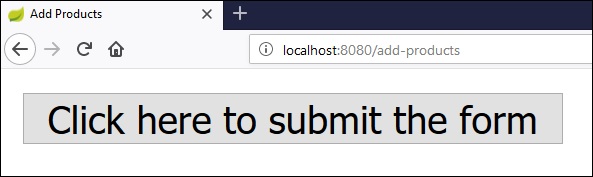
现在,单击按钮Click here to submit the form ,您可以看到如下所示的结果 -
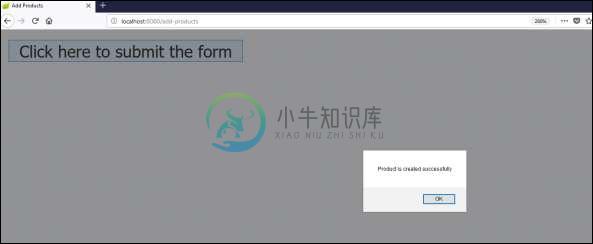
现在,点击查看产品网址,查看创建的产品。
http://localhost:8080/view-products
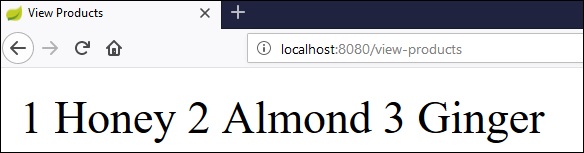
Angular JS
要使用Angular JS来使用API,您可以使用下面给出的示例 -
使用以下代码创建Angular JS Controller以使用GET API - http://localhost:9090/products -
angular.module('demo', [])
.controller('Hello', function($scope, $http) {
$http.get('http://localhost:9090/products').
then(function(response) {
$scope.products = response.data;
});
});
使用以下代码创建Angular JS Controller以使用POST API - http://localhost:9090/products -
angular.module('demo', [])
.controller('Hello', function($scope, $http) {
$http.post('http://localhost:9090/products',data).
then(function(response) {
console.log("Product created successfully");
});
});
Note - Post方法数据表示以JSON格式创建产品的Request主体。