格式化文本( Formatting Text)
优质
小牛编辑
125浏览
2023-12-01
可以使用XSLFTextRun类的方法格式化演示文稿中的文本。 为此,您必须通过选择其中一个幻灯片布局来创建XSLFTextRun类对象,如下所示 -
//create the empty presentation
XMLSlideShow ppt = new XMLSlideShow();
//getting the slide master object
XSLFSlideMaster slideMaster = ppt.getSlideMasters()[0];
//select a layout from specified list
XSLFSlideLayout slidelayout = slideMaster.getLayout(SlideLayout.TITLE_AND_CONTENT);
//creating a slide with title and content layout
XSLFSlide slide = ppt.createSlide(slidelayout);
//selection of title place holder
XSLFTextShape body = slide.getPlaceholder(1);
//clear the existing text in the slide
body.clearText();
//adding new paragraph
XSLFTextParagraph paragraph = body.addNewTextParagraph();
//creating text run object
XSLFTextRun run = paragraph.addNewTextRun();
您可以使用setFontSize()设置演示文稿中文本的字体大小。
run.setFontColor(java.awt.Color.red);
run.setFontSize(24);
以下代码段显示如何将不同的格式样式(粗体,斜体,下划线,删除线)应用于演示文稿中的文本。
//change the text into bold format
run.setBold(true);
//change the text it to italic format
run.setItalic(true)
// strike through the text
run.setStrikethrough(true);
//underline the text
run.setUnderline(true);
要在段落之间使用换行符,请使用XSLFTextParagraph类的addLineBreak() ,如下所示 -
paragraph.addLineBreak();
以下是使用上述所有方法格式化文本的完整程序 -
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.xslf.usermodel.SlideLayout;
import org.apache.poi.xslf.usermodel.XMLSlideShow;
import org.apache.poi.xslf.usermodel.XSLFSlide;
import org.apache.poi.xslf.usermodel.XSLFSlideLayout;
import org.apache.poi.xslf.usermodel.XSLFSlideMaster;
import org.apache.poi.xslf.usermodel.XSLFTextParagraph;
import org.apache.poi.xslf.usermodel.XSLFTextRun;
import org.apache.poi.xslf.usermodel.XSLFTextShape;
public class TextFormating {
public static void main(String args[]) throws IOException {
//creating an empty presentation
XMLSlideShow ppt = new XMLSlideShow();
//getting the slide master object
XSLFSlideMaster slideMaster = ppt.getSlideMasters()[0];
//select a layout from specified list
XSLFSlideLayout slidelayout = slideMaster.getLayout(SlideLayout.TITLE_AND_CONTENT);
//creating a slide with title and content layout
XSLFSlide slide = ppt.createSlide(slidelayout);
//selection of title place holder
XSLFTextShape body = slide.getPlaceholder(1);
//clear the existing text in the slide
body.clearText();
//adding new paragraph
XSLFTextParagraph paragraph = body.addNewTextParagraph();
//formatting line 1
XSLFTextRun run1 = paragraph.addNewTextRun();
run1.setText("This is a colored line");
//setting color to the text
run1.setFontColor(java.awt.Color.red);
//setting font size to the text
run1.setFontSize(24);
//moving to the next line
paragraph.addLineBreak();
//formatting line 2
XSLFTextRun run2 = paragraph.addNewTextRun();
run2.setText("This is a bold line");
run2.setFontColor(java.awt.Color.CYAN);
//making the text bold
run2.setBold(true);
paragraph.addLineBreak();
//formatting line 3
XSLFTextRun run3 = paragraph.addNewTextRun();
run3.setText(" This is a striked line");
run3.setFontSize(12);
//making the text italic
run3.setItalic(true);
//strike through the text
run3.setStrikethrough(true);
paragraph.addLineBreak();
//formatting line 4
XSLFTextRun run4 = paragraph.addNewTextRun();
run4.setText(" This an underlined line");
run4.setUnderline(true);
//underlining the text
paragraph.addLineBreak();
//creating a file object
File file = new File(“TextFormat.pptx”);
FileOutputStream out = new FileOutputStream(file);
//saving the changes to a file
ppt.write(out);
out.close();
}
}
将上面的代码保存为TextFormating.java ,然后从命令提示符编译并执行它,如下所示 -
$javac TextFormating.java
$java TextFormating
它将编译并执行以生成以下输出 -
Formatting completed successfully
带有格式化文本的幻灯片如下所示 -
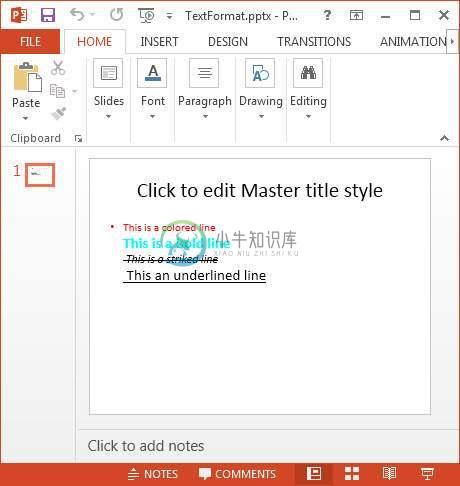